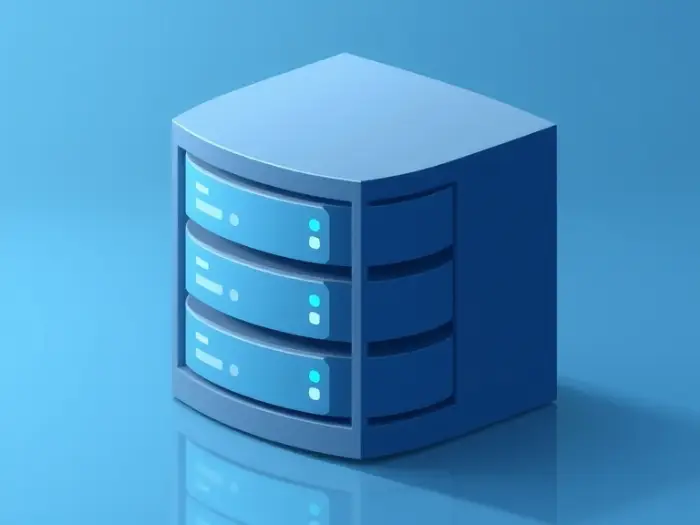
In the world of web development, understanding Web Storage is essential for managing client-side data efficiently. Among the various options available, Session Storage, Local Storage, Cookies, and IndexedDB each offer unique benefits. While Cookies are often used for tracking and session management, Local Storage and Session Storage provide different ways to persist data during a user’s interaction with a site. IndexedDB, on the other hand, offers a powerful solution for handling large amounts of structured data. Understanding the distinctions between these technologies will help you choose the best option for your project.
Session Storage
Session Storage is a feature of Web Storage, a client-side storage API available in modern browsers. It allows data to be stored temporarily during the user’s browsing session.
Features:
- Limited capacity between 5 to 10MB.
- Data is retained only for the duration of the browser session.
// Save data to session storage
sessionStorage.setItem('name', 'john');
// Get data from session storage
let data = sessionStorage.getItem('name');
Local Storage
Local Storage is another feature of Web Storage, similar to Session Storage, but with a different lifespan. Unlike Session Storage, data persists even after the browser is closed or the computer is restarted. The data is retained indefinitely until explicitly deleted by the application or the user.
const user = {
name: 'john',
age: 30
};
localStorage.setItem('user', JSON.stringify(user));
Cookies
Cookies are a method for storing small amounts of client-side data, sent by the server and stored by the browser. They are used for various purposes, such as managing sessions, storing user preferences, and tracking users across multiple sites.
Features:
- Cookies can have a lifespan defined by the
Expires
orMax-Age
attribute. - Limited capacity of around 4 KB per cookie, with a maximum number of cookies per domain varying by browser.
document.cookie = "sessionId=38afes7a8; expires=Tue, 19 Jan 2038 03:14:07 GMT";
// Reading cookies
let cookies = document.cookie.split(';');
let sessionId = cookies.find(cookie =>
cookie.includes('sessionId'));
IndexedDB
IndexedDB is a powerful client-side storage API that allows storing large amounts of structured data. Unlike cookies or Local/Session Storage, IndexedDB offers a much larger storage capacity and is designed to handle more complex data.
Features:
- Data stored in IndexedDB persists indefinitely until explicitly deleted.
- The storage capacity is generally much larger than Local/Session Storage.
// Open database
const request = indexedDB.open('notes', 1);
request.onerror = () => console.error('Database failed');
request.onsuccess = () => {
// Get database object
const db = request.result;
};