JavaScript keeps evolving to offer developers more powerful and readable tools. One of the most useful recent additions is the Nullish Coalescing Operator (??
), introduced in ES2020. This operator may seem subtle at first, but it allows for cleaner, safer, and more intentional code. In this article, weโll dive into what this operator does, how it works, and when to use it.
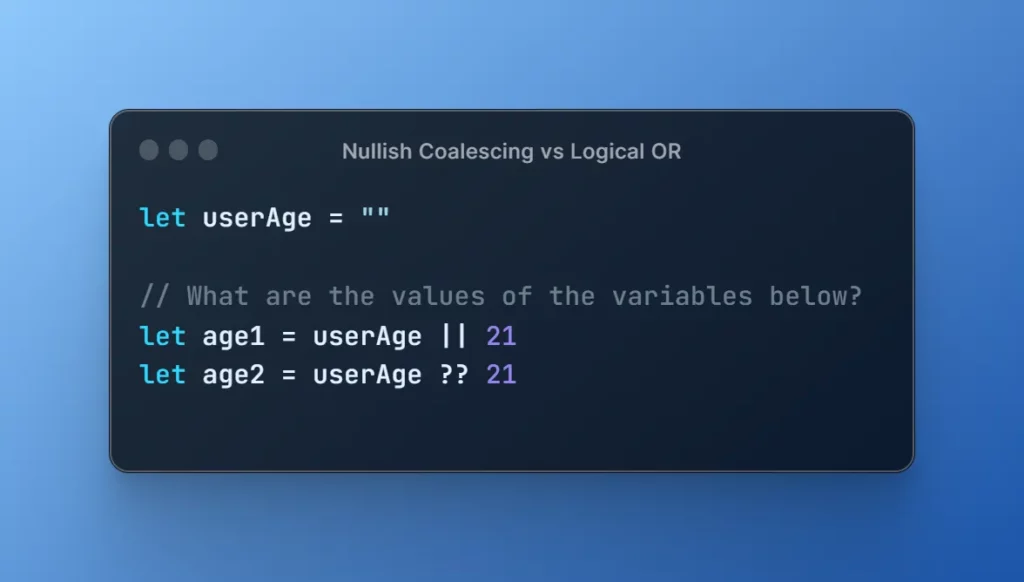
๐ What is ??
Exactly?
The Nullish Coalescing Operator (??
) is a logical operator that returns the right-hand operand if the left-hand operand is null
or undefined
, and returns the left-hand operand otherwise.
let result = a ?? b;
In plain terms:
๐ If a
is null
or undefined
, then result
is b
. Otherwise, result
is a
.
๐ก How is it Different from ||
(Logical OR)?
Great question! This is where ??
really shines.
The ||
operator returns the first falsy value it encounters, which includes 0
, ''
(empty string), false
, and more.
However, ??
only considers null
and undefined
to be โnullishโ.
๐ฌ Comparison Example:
let value = 0;
console.log(value || 42); // Outputs 42
console.log(value ?? 42); // Outputs 0
โ
value || 42
returns 42
because 0
is falsy.
โ
value ?? 42
returns 0
because 0
is not null
or undefined
.
๐ง When Should You Use ??
?
Use ??
when you want to set a default value only if a variable is null
or undefined
, not just any falsy value.
โ A Common Use Case:
function getUserName(name) {
return name ?? "Guest";
}
console.log(getUserName(null)); // "Guest"
console.log(getUserName(undefined)); // "Guest"
console.log(getUserName("Alice")); // "Alice"
console.log(getUserName("")); // ""
Here, an empty string is treated as a valid value. It wonโt be replaced by “Guest” when using ??
.
โ ๏ธ A Word of Caution
๐ Avoid Mixing ??
with ||
or &&
Without Parentheses:
JavaScript will throw a SyntaxError if you combine these operators without clear grouping:
// โ SyntaxError
let x = a || b ?? c;
โ Always use parentheses to make your intentions clear:
let x = (a || b) ?? c;
๐ฏ Conclusion
The Nullish Coalescing Operator (??
) is a small addition with a big impact. It allows you to handle null
or undefined
values cleanly, without interfering with other falsy values like 0
or false
. If you want more readable and precise code, this operator is definitely worth using!
๐ ๏ธ Quick Recap
??
only handlesnull
andundefined
.- Great for default values.
- More precise than
||
in many cases. - Watch out for mixing with other logical operators without parentheses!