State management in React apps can often become a major headache. Between Redux, Recoil, Jotai, or the Context API, choosing the right solution can be overwhelming.
But one tool has recently been gaining popularity in the frontend community: Zustand. Lightweight, efficient, and incredibly easy to use, Zustand allows you to create global state without the hassle, and without sacrificing performance.
In this article, we’ll explore how it works, how to integrate it into a React project, and why it might just become your new favorite state management library.
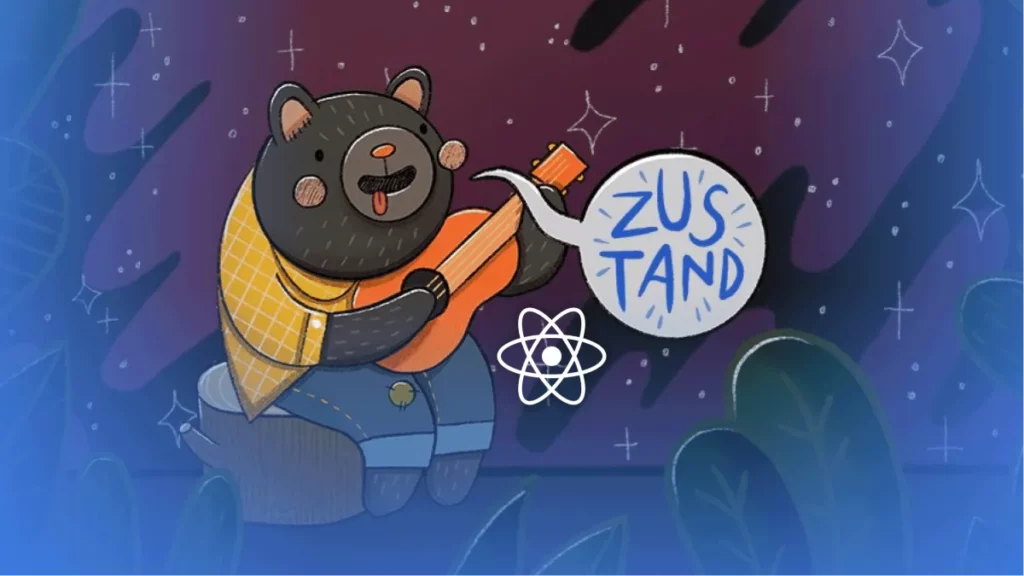
What is Zustand?
Zustand (which means state in German) is a lightweight JavaScript library for state management in React applications. It’s developed by the team at Poimandres, the creators of react-three-fiber
.
Key Features:
- No need for context providers
- Simple API based on hooks
- Built-in selectors to prevent unnecessary re-renders
- Middleware support (persist, immer, devtools, etc.)
- Async/await friendly
How Does Zustand Work?
It works by creating a global store that any React component can access through a custom hook.
// store.js
import { create } from 'zustand'
const useCounterStore = create((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
reset: () => set({ count: 0 }),
}))
In a React component:
// CounterComponent.js
import useCounterStore from './store'
const Counter = () => {
const { count, increment, decrement, reset } = useCounterStore()
return (
<div>
<h2>Counter: {count}</h2>
<button onClick={increment}>+1</button>
<button onClick={decrement}>-1</button>
<button onClick={reset}>Reset</button>
</div>
)
}
No Provider, no complex setup. Just use a hook and you’re good to go!
Zustand Architecture Explained
ustand is designed to create a reactive store using custom hooks. Here are its core concepts:
1. Global store
All your state is centralized in a single, shared store. Every component that calls useStore()
accesses the same data.
2. State updates via set()
Updates the store using the set()
function, which automatically triggers the re-renders where needed.
3. Optimized rendering
you can select only the parts of the state you need, so your components re-render only when that specific part changes.
const count = useCounterStore((state) => state.count)
4. Pure React experience
Unlike Redux, there’s no mapStateToProps
or HOCs. Zustand fits seamlessly into React’s modern hook-based architecture.
Advanced Zustand Usage
1. Async API Calls
const useUserStore = create((set) => ({
user: null,
fetchUser: async () => {
const res = await fetch('/api/user')
const data = await res.json()
set({ user: data })
},
}))
ou can then call fetchUser()
inside a useEffect
or on button click.
2. Using Middleware (persist, immer, devtools)
import { create } from 'zustand'
import { persist } from 'zustand/middleware'
const useAuthStore = create(persist((set) => ({
isLoggedIn: false,
login: () => set({ isLoggedIn: true }),
logout: () => set({ isLoggedIn: false }),
}), {
name: 'auth-storage', // saved in localStorage
}))
🎯 With persist
, your state survives page reloads.
Zustand is a modern, simple, and efficient solution for managing global state in React applications. With its intuitive API, high performance, and seamless integration into React’s hooks system, it’s a serious contender to Redux for modern projects.
Officiel site : https://zustand.docs.pmnd.rs/getting-started/introduction