When building applications with React, state management quickly becomes a challenge as multiple components need to share the same data. This is where React Context comes in. In this article, you will learn:
- Why state management is crucial in React
- What prop drilling is and its downsides
- How to use the React Context API step-by-step
- Performance optimizations and best practices
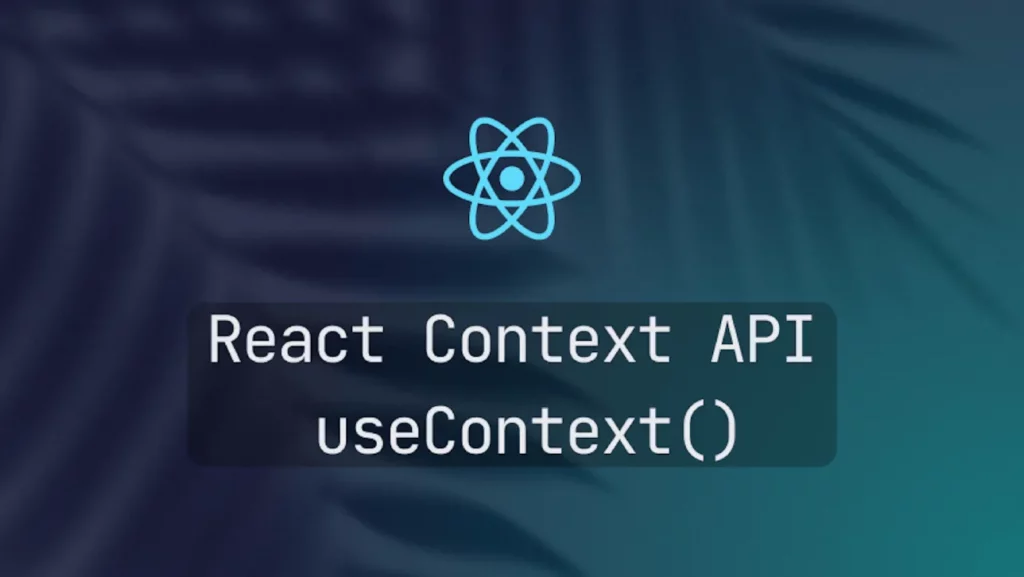
Why State Management Is Essential in React
React is a JavaScript library focused on building dynamic user interfaces. To make your components interactive, you need to manage state — that means storing and updating data like user input, themes, language preferences, or API data.
On a small scale, useState
is enough. But as your app grows, some state needs to be shared across multiple components. That’s when complexity arises.
The Problem With Prop Drilling
Prop drilling is when you pass props through several layers of components, even if only one of them actually needs it. Example:
<App>
<Parent>
<Child>
<GrandChild theme={theme} />
</Child>
</Parent>
</App>
In this example, theme
is passed from the top-level component down to GrandChild
, even though only GrandChild
uses it. This clutters your code and makes it harder to maintain.
The React Context API: A Clean Solution
The React Context API allows you to share data across the component tree without passing props manually at every level. Here’s how to use it step by step:
1. Create a Context
import { createContext } from "react";
export const ThemeContext = createContext();
2. Create a Provider
import React, { useState } from "react";
export const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState("light");
return (
<ThemeContext.Provider value={{ theme, setTheme }}>
{children}
</ThemeContext.Provider>
);
};
3. Use Context in Your Components
import { useContext } from "react";
import { ThemeContext } from "./ThemeContext";
const ThemedComponent = () => {
const { theme } = useContext(ThemeContext);
return <div className={`app-container ${theme}`}>Hello Theme!</div>;
};
4. Wrap Your App With the Provider
import ReactDOM from "react-dom/client";
import { ThemeProvider } from "./ThemeContext";
import App from "./App";
ReactDOM.createRoot(document.getElementById("root")).render(
<ThemeProvider>
<App />
</ThemeProvider>
);
Performance Optimizations & Best Practices
While React Context is powerful, improper use can lead to unnecessary re-renders.
✅ Best Practices:
- Don’t put everything into a single context. Create dedicated contexts:
AuthContext
,ThemeContext
, etc. - Memoize the provider value using
useMemo
to avoid re-renders:
const value = useMemo(() => ({ theme, setTheme }), [theme]);
- Use
React.memo
oruseContextSelector
(via theuse-context-selector
library) for performance-sensitive components.
The React Context API is a powerful tool that helps you avoid prop drilling and manage global state more easily in your React apps. When used properly and combined with performance best practices, it leads to cleaner, modular, and more efficient code.