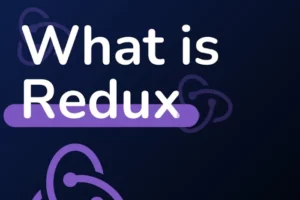
Overview of Redux
Store:
The store holds all of your application’s state in a single, centralized location. Think of it as a JavaScript object representing your entire application’s state.
Actions:
Actions, simple JavaScript objects, describe events in your application. Components or other parts of your application can dispatch actions to trigger changes in the state.
Reducers:
Reducers are functions that define how actions transform the application’s state. They accept the current state and an action as inputs and return the updated state.
Middleware:
Middleware enhances Redux with additional functionality. It can intercept actions, handle asynchronous tasks, and modify actions before they reach reducers.
View:
Your UI components, usually React-based, subscribe to the Redux store, reading data from it and re-rendering when the data changes.
Example Flow in Redux
1- Install Redux and React-Redux
npm install redux react-redux
2- Create a Redux Store with a Reducer
import { createStore } from 'redux';
// Define initial state
const initialState = {
count: 0 // Assuming count is a property of your state
};
// Define the reducer function
const reducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
case 'DECREMENT':
return { ...state, count: state.count - 1 };
default:
return state;
}
};
// Create the Redux store
const store = createStore(reducer);
export default store;
3- Use the Store in a React Component
import React from 'react';
import { connect } from 'react-redux';
class Counter extends React.Component {
render() {
return (
<div>
<p>Count: {this.props.count}</p>
<button onClick={this.props.increment}>Increment</button>
<button onClick={this.props.decrement}>Decrement</button>
</div>
);
}
}
// Define mapStateToProps to map state to props
const mapStateToProps = (state) => ({
count: state.count
});
// Define mapDispatchToProps to map actions to props
const mapDispatchToProps = (dispatch) => ({
increment: () => dispatch({ type: 'INCREMENT' }),
decrement: () => dispatch({ type: 'DECREMENT' })
});
// Connect the component to the Redux store
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
4- Wrap the App with the Redux Provider:
import React from 'react';
import { Provider } from 'react-redux';
import store from './store'; // Corrected the path to store
import Counter from './Counter';
function App() {
return (
<Provider store={store}>
<div className="App">
<Counter />
</div>
</Provider> // Corrected the closing tag
);
}
export default App;
Conclusion
In this setup, the Redux store manages the state, and the Counter
component connects to the store using connect
from react-redux
. Clicking the “Increment” or “Decrement” buttons dispatches actions to the store, updating the state and causing the component to re-render accordingly.