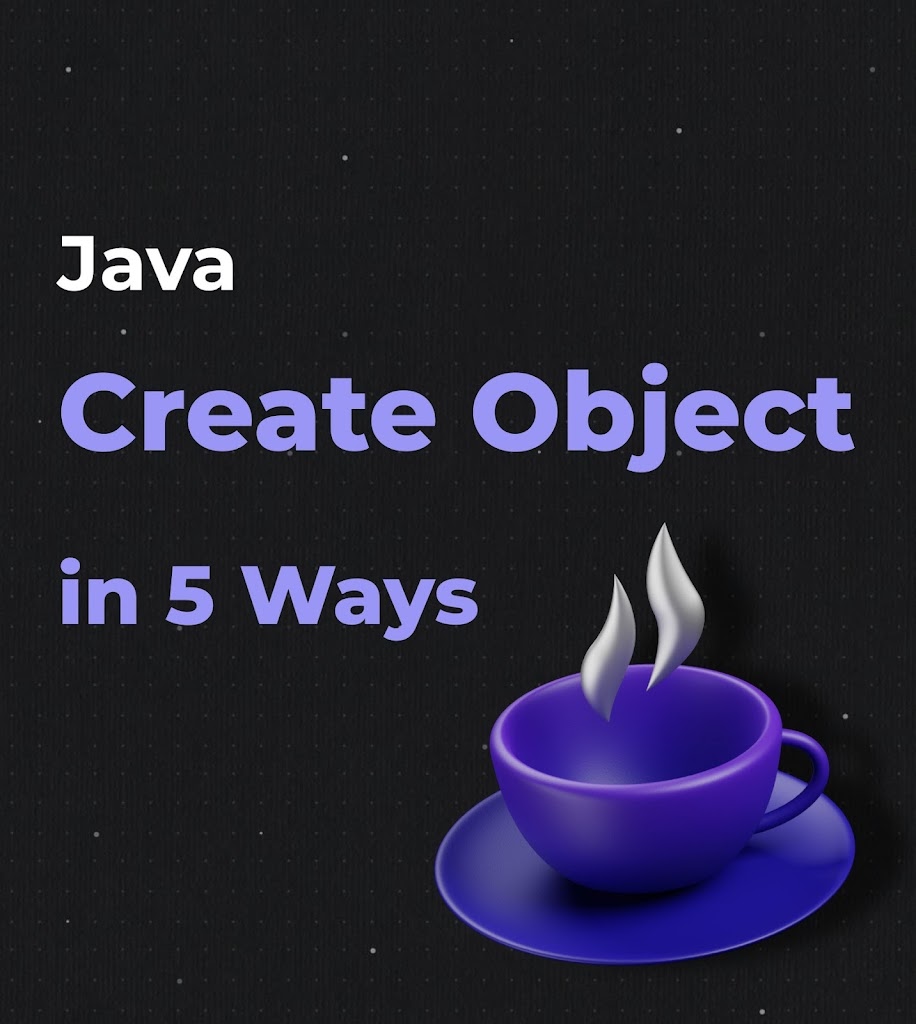
1. Using a new keyword.
– It’s the most popular one. We create an object by using a new operator followed by a constructor call.
Exemple :
class ClassA{
}ClassA obj = new ClassA()
2. newinstance() method
– Using the newInstance() method of class “Class”
Exemple :
class ClassA {
}
ClassA obj = ClassA.class.newInstance();
3. newinstance() method – 2
– Using the newInstance() method in class “Constructor”.
Exemple :
class ClassA {
}Constructor<ClassA> obj = ClassA.class.getConstructor().newInstance();
– Both the previous ways (Shown in 2 and 3), are known as reflective ways of creating objects.
Fun-fact:
– Class’s newInstance() method internally uses Constructor’s newInstance() method.
4. clone method
– Using “Object” class clone() method. The clone() method creates a copy of an existing object.
– The clone) method is part of the “Object” class which returns a clone object.
– Example:
public class ClassA implements Cloneable {
protected Object clone() throws CloneNotSupportedException {//.....}}
ClassA obj1 = new ClassA( );
ClassA obj2 = (ClassA)obj1.clone();
– Always Remember:
- The “Cloneable” interface is implemented.
- The clone() method must be overridden with other classes.
- Inside the clonel() method, the class must call super.clone().
Learn more : Cloning in Java: Understanding Shallow Copy and Deep Copy
5. Deserialization
– When we deserialize any object then JVM creates a new object internally.
For this, we need to implement the Serializable interface.
– Example:
import java.io.*;
public class ClassA implements Serializable {
// Attributs et méthodes de la classe
}
public class SerializationExample {
public static void main(String[] args) {
// Création d'un objet pour la sérialisation
ClassA classA = new ClassA();
// Sérialisation
try (ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("class.obj"))) {
out.writeObject(classA);
} catch (IOException e) {
e.printStackTrace();
}
// Désérialisation
ClassA deserialClassA = null;
try (ObjectInputStream in = new ObjectInputStream(new FileInputStream("class.obj"))) {
deserialClassA = (ClassA) in.readObject();
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}