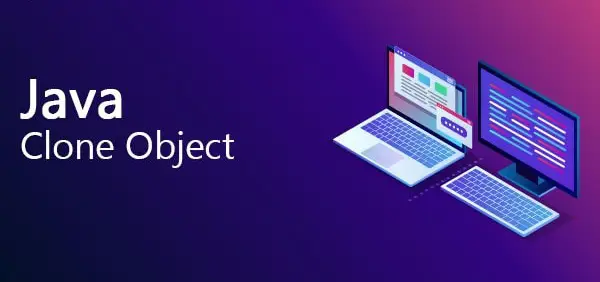
Cloning is a fundamental feature in Java that enables the creation of an exact copy of an object in memory. This operation relies on the clone() method of the Object class, which is designed to duplicate objects. However, using it requires a precise understanding of its functionality and limitations. In Java, cloning can be performed using either Shallow Copy or Deep Copy techniques, each with distinct behavior. Cloning Java objects through a shallow copy only duplicates the object’s primitive attributes, while Deep Copy ensures that all referenced objects are also cloned, preventing shared references between the original and copied objects. Understanding both Shallow Copy and Deep Copy is essential for effective cloning in Java.
What is Cloning in Java?
Cloning in Java refers to creating a copy of an existing object without invoking the object’s constructor. The clone()
method performs this operation but comes with key nuances:
- It duplicates the object’s primitive attributes.
- It does not copy the content of reference-type attributes, instead referencing the same objects or arrays in memory.
Practical Example: How the clone()
Method Works
Consider a class A
with two attributes:
- An integer attribute
n
. - An attribute
t
, which is a reference to an array or another object.
Here’s what happens during cloning:
A aCopie = a.clone();
- For primitive types: The attribute
n
is copied with its current value. Any modification ofn
in the clone will not affectn
in the original object. - For reference types: The attribute
t
continues to point to the same object or array as in the original instance. Consequently, any change to the content of the object referenced byt
will also affect the original.
Requirements and Restrictions
The clone()
method of the Object
class imposes several constraints to ensure proper cloning:
Cloneable
Interface:
A class must declare that it implements the java.lang.Cloneable
interface. For example:
public class A implements Cloneable {
// Class code
}
Without this declaration, attempting to clone an object will throw a CloneNotSupportedException
, indicating that the object is not eligible for cloning.
- Exception Handling: The
clone()
method requires exception handling to avoid crashes:
try { A aCopy = a.clone(); }
catch (CloneNotSupportedException e) { e.printStackTrace(); }
Limitations of Cloning with clone()
While convenient, the clone()
method has significant limitations:
- Shallow Cloning: By default,
clone()
performs a shallow copy, duplicating only the primitive attributes. Shared references to objects or arrays can lead to unexpected behavior. - Complex Customization: If a class contains references to complex objects, it is necessary to override the
clone()
method to implement deep cloning. This involves cloning the referenced objects as well.
Deep Cloning: An Alternative Solution
Deep cloning duplicates not only primitive attributes but also the content of referenced objects or arrays. This ensures no shared references exist between the original object and its copy.
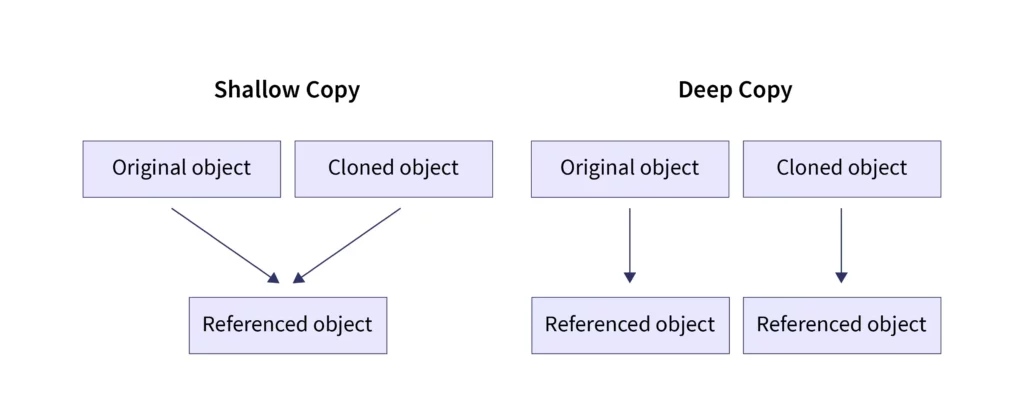
Here’s an example of a class containing an integer array, with an overridden clone()
method for deep cloning:
public class EntierA implements Cloneable {
private int[] array;
// Constructor
public EntierA(int[] array) {
this.array = array;
}
// Getter for the array
public int[] getArray() {
return array;
}
@Override
protected Object clone() throws CloneNotSupportedException {
// Call the Object class's clone() method for shallow copy
EntierA copy = (EntierA) super.clone();
// Perform deep cloning for the array
if (this.array != null) {
copy.array = new int[this.array.length];
System.arraycopy(this.array, 0, copy.array, 0, this.array.length);
}
return copy;
}
// Main method to test cloning
public static void main(String[] args) {
try {
// Create an original object
int[] originalArray = {1, 2, 3, 4};
EntierA original = new EntierA(originalArray);
// Clone the object
EntierA copy = (EntierA) original.clone();
// Modify the copy to verify object independence
copy.getArray()[0] = 99;
// Display results
System.out.println("Original array: " + java.util.Arrays.toString(original.getArray()));
System.out.println("Copied array: " + java.util.Arrays.toString(copy.getArray()));
} catch (CloneNotSupportedException e) {
e.printStackTrace();
}
}
}
- The
super.clone()
method is used to copy primitive attributes. - The array
array
is recreated by allocating new memory space. - The
System.arraycopy()
method copies the values from the original array to the copy’s array. This ensures the copy does not share the original array’s reference.
After cloning, modifying the copy’s array does not affect the original, demonstrating the effectiveness of deep cloning.