In modern front-end development, managing HTTP requests is becoming increasingly complex. Between loading states, error handling, caching, synchronization, and interdependent requests, a simple fetch
or even axios
is often no longer sufficient. This is where Alova.js positions itself as a reactive, complete, and elegant solution.
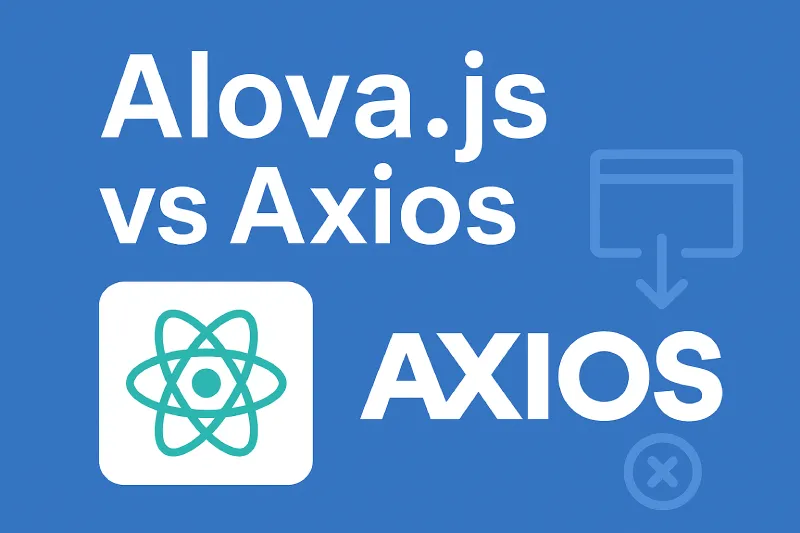
β¨ What is Alova.js?
Alova.js is a JavaScript library designed to handle HTTP requests reactively, with native integration into modern frameworks like React, Vue, and Svelte. Its goal: to centralize network logic, automate state management (loading
, data
, error
), and reduce boilerplate in UI components.
The name stands for Abstract Layer of Various API, and its architecture reflects that: abstraction, modularity, and extensibility.
βοΈ Main Features
1. Built-in Reactive Requests
Alova provides hooks like useRequest
, useWatcher
, usePagination
, etc., which return reactive states directly usable in your components.
const { loading, data, error } = useRequest(() =>
alova.Get('/posts'), { immediate: true });
2. Automatic State Management
No need to write repetitive useState
or useEffect
: Alova handles it for you.
3. Smart Caching
Each request can cache data with rules for expiration or refresh.
4. Conditional and Dependent Requests
With useWatcher
, you can trigger requests based on state changes or dependencies.
useWatcher(() => alova.Get(`/users/${userId}`), [userId]);
5. Centralized Configuration
Headers, transformations, errors, etc. are defined once in the alova
instance.
const alova = createAlova({
baseURL: 'https://api.example.com',
statesHook: ReactHook,
beforeRequest(method) {
method.config.headers.Authorization = 'Bearer token';
},
});
π§ Why Choose Alova.js?
Classic Axios Example
useEffect(() => {
axios.get('/posts')
.then(res => setData(res.data))
.catch(setError)
.finally(() => setLoading(false));
}, []);
With Alova.js
const { loading, data, error } = useRequest(() =>
alova.Get('/posts'), { immediate: true });
Result: Less code, better readability, automatic states.
π Ideal For
- SPAs built with React/Vue/Svelte
- Projects that need caching and pagination
- Apps requiring automatic loading/error/data handling
π¦ Installation
npm install alova
# or
yarn add alova
React Setup
import ReactHook from 'alova/react';
import { createAlova } from 'alova';
const alova = createAlova({
baseURL: '/api',
statesHook: ReactHook
});
π Resources
π Conclusion
Alova.js is not just another HTTP client. Itβs a modern, efficient, and developer-friendly tool to manage requests in modern front-end apps. Compared to Axios, it offers better reactivity, less boilerplate, and more scalability for real-world use cases.