In Java, comparing two numbers seems straightforward: 1 == 1 returns true, so 128 == 128 should as well, right? However, Java has a little surprise for us here.
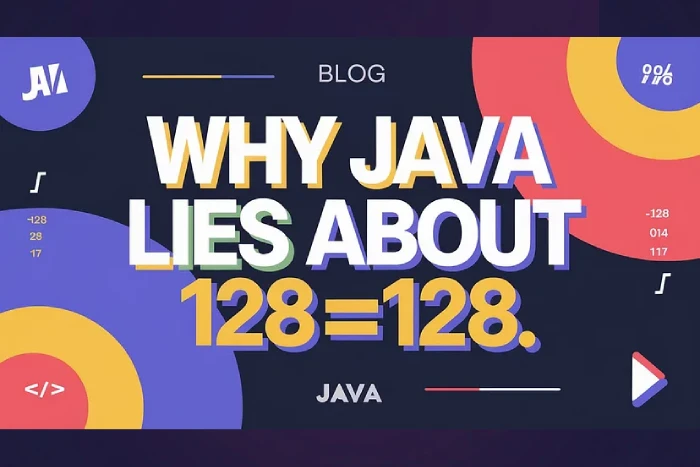
The Impact of Caching on Comparisons in Java
In Java, the Integer class uses a caching mechanism to optimize memory usage, mainly by avoiding unnecessary object creation. This system caches all Integer instances representing values between -128 and 127. Therefore, when you assign values within this range, Java uses the same object in memory for each instance.
Here’s a concrete example:
Integer x = 1;
Integer y = 1;
System.out.println(x == y); // true
In this example, Java points x
and y
to the same memory reference to save space. The comparison ==
returns true
because both variables refer to the same object.
Outside the Range: Creating New Objects
However, when dealing with values outside the -128 to 127 range, Java does not reuse the same objects. Instead, it creates a new instance for each assignment. So:
Integer a = 128;
Integer b = 128;
System.out.println(a == b); // false
In this case, Java creates two distinct objects for a
and b
because 128 is outside the cached range. As a result, the comparison ==
returns false
because a
and b
do not point to the same reference.
Difference Between ==
and .equals()
In Java, it’s crucial to understand that ==
compares references, meaning the memory location of the objects, whereas .equals()
compares the values of the objects themselves. For instance:
Integer a = 128;
Integer b = 128;
System.out.println(a.equals(b)); // true
Here, a.equals(b)
returns true
because it compares the values of the objects, not their references.
The Cache Range and Memory Optimization
By default, Java caches Integer
values between -128 and 127. This range can be modified using the JVM argument -XX:AutoBoxCacheMax=<size>
. For example, setting -XX:AutoBoxCacheMax=200
would allow caching all values between -128 and 200.
In summary, in Java, comparing Integer
objects with ==
can yield unexpected results due to reference management and caching. Use .equals()
to compare Integer
values, and be mindful of the caching effects, especially when working with values outside the -128 to 127 range.